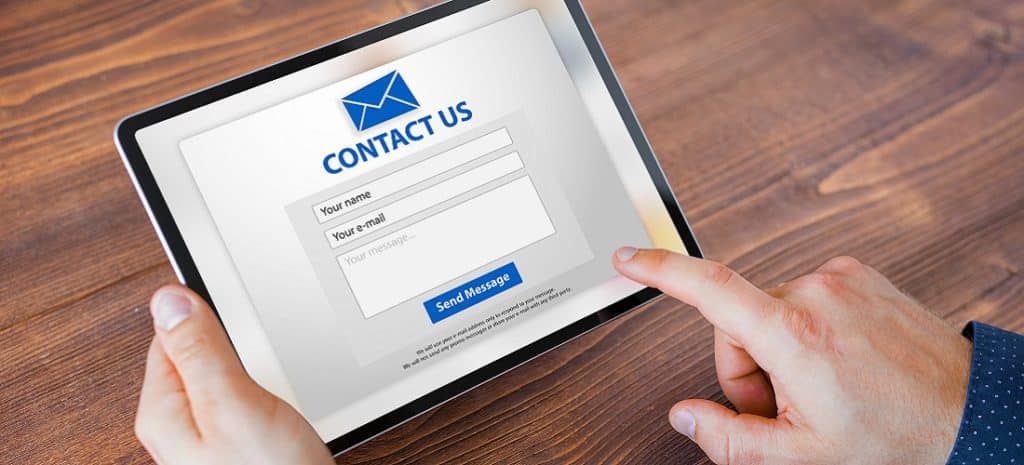
How to Create a Form Using WordPress (In 4 Steps)
Online forms come in all shapes and sizes. They offer a convenient way to gather information and feedback from your audience. Knowing how to create a form using WordPress can help you kickstart your marketing efforts and better understand your customers.
Luckily, plugins make it easy to create and embed forms anywhere on your WordPress site. With pre-made templates, you can make forms in a matter of minutes and manage the data from a convenient dashboard. What you do with the information you gather is up to you!
In this article, we’ll explain why you may want to use WordPress forms. Then, we’ll provide you with a guide for how to create them. We’ll also show you how you can utilize them for marketing and other purposes. Let’s get started!
Why You May Want to Use a Form in WordPress
Forms provide a highly useful way to collect data from your visitors. They can be used in a number of ways. Some common examples include:
- Contact forms
- Account registration
- Surveys
- Quizzes
- Payment forms
Essentially, a form presents users with a series of fillable fields where they can enter and submit data in response to prompts. This data is then sent to a server where it can be processed for review or direct automation.
Even though you can use forms to collect data for any number of reasons, one of the most frequently-seen examples is when building an email list. You can generate an email list using contact forms, account registrations, and even checkout forms:
Since WordPress is a wildly popular platform, it boasts a wide selection of plugins that can assist with designing and implementing forms. These extensions usually include pre-made templates and a drag-and-drop interface.
Moreover, they usually allow you to customize your own entry fields and prompts. These tools will also handle processing form submissions so you can easily review and make use of the results.
All in all, forms offer a simple but effective method for connecting with your audience. Using the voluntary feedback they provide can be crucial for marketing, website development, and even day-to-day functions like account creation and payment processing.
How to Create a Form Using WordPress (In 4 Steps)
While there are many different methods for creating and implementing forms, we will cover a representative example using a popular and free form builder. Just follow these four steps to create your own WordPress forms quickly and easily!
Step 1: Install the Ninja Forms plugin
Ninja Forms is one of the most popular form builders available on WordPress. It’s free to download and has everything you need to create a variety of basic forms. Premium upgrades with advanced features are available, but the free version has a lot to offer.
To install Ninja Forms, just hover over the Plugins tab on the left-hand side of your WordPress dashboard and select Add New. Then, type “ninja forms” into the search bar. Once you locate the plugin, you can install and activate it as you normally would:
After that, you should see a new tab on the left-hand side of your main dashboard called Ninja Forms. This is where you will design and manage your forms.
Step 2: Creating a Form
Creating a form with Ninja Forms is a breeze. To get started, go to the new Ninja Forms tab on your dashboard and select Add New.
You will then be presented with a number of templates you can use to expedite the process. These templates cover most common needs, so simply make your selection or use the search bar to look for something specific:
A template will automatically have most of the fields you need built-in. Still, you can easily edit and remove these fields by clicking on them in the main display window. You’ll then see more detailed editing options on the right:
To add new fields, simply click on the + symbol at the bottom right of the screen. This will bring up a selection of common fields that you can add to your form:
You can easily drag and drop these new elements onto the form, which acts much like the WordPress block editor. If you can’t find a template that’s appropriate for your needs, you can easily create a new form from scratch using the blank template.
Step 3: Embed Forms on Your Site
Once you have created a form, you can embed it on your site simply by choosing the Ninja Form block from your block editor screen. You will find it within the collection of Text blocks, or you can locate it using the search bar:
After placing the block on a page or in a post, you’ll be able to select which form you want to embed from the dropdown menu. This will automatically load that form into the block location.
Once you’ve selected your form, then you’ll need to make sure to publish or update your post or page. This way, your changes will go live on your website.
Now, all your visitors have to do is complete the required fields and press the submit button to record their response. It’s as easy as that!
Step 4: Monitor the Results
Once your Ninja Form is embedded on your site, it will start collecting user responses. That is, as long as people are filling out your form. To view your new form data, go to Ninja Forms > Submissions in your WordPress dashboard.
Here you will need to select which form you want to review from the dropdown menu at the top of the page. The results will be loaded below, allowing you to review, filter, and even download submission data:
Depending on the fields in the form, the submissions data will automatically change to match it. For example, a simple contact form will include a name and email address, while a survey will have a variety of different questions and answers. You can click on each submission entry for full details.
How to Integrate WordPress Forms with Your Email Marketing Strategy
Forms offer a great way to collect contact information from visitors and potential customers. You can easily use the results from your forms in email marketing by downloading the data to use elsewhere.
For example, you can copy the data as a CSV file to use in a spreadsheet or directly import it into email software such as Mailchimp.
To do this, go to the Submissions page and choose the form whose data you want to collect from the dropdown menu at the top of the screen. Then, click the Download All Submissions button at the bottom of the page to receive your CSV file:
You can even set up a more direct Mailchimp integration to automate the process of collecting email addresses and adding them to an email campaign.
Another option is to add actions directly to Ninja Forms which can automatically send out an email to an entered address when the form is submitted. This feature is often used to send confirmation emails, but can also be used as the first step for email marketing.
To do this, click on the Emails & Actions tab when creating a form and select which message you want to modify. In this example we are editing the email confirmation and sending it to the email entered in the form:
You can design this email however you’d like and it will automatically send when the form is submitted.
Collecting other useful information within your contact form, such as customer interests or demographics, can provide you with useful insights you can then use to curate more targeted marketing emails. There are limitless possibilities, so you shouldn’t feel constrained just to what is included in the templates.
Other Ways to Use WordPress Forms
Now let’s explore additional ways you can use WordPress forms to improve your site and connect with your users.
Surveys
Online surveys are a fun way to learn more about your visitors or get feedback about your company. You can use them strictly for market research or to influence business decisions.
Surveys can also just be a fun way to connect with your audience and get to know them better. You may want to ask questions like “What is your favorite color?” or “What should I name my new cat?” for no other reason than entertainment and community building.
Contests
Contests are another great use of forms. You can use them to collect contact information or even meaningful contributions.
For instance, a simple contest may ask people to sign up for your email list for a chance to win a prize. When the contest is finished, you can simply choose a submission from the dashboard at random. Another example might be asking your community to submit a logo design for a chance to win a reward. In this case, you would need to review the submissions one-by-one and select a winner.
The great thing about contests is that they may motivate users to fill out a form they would have otherwise scrolled past. After all, people will be much more likely to visit your site when they have the potential to win something.
Contact Forms
Contact forms offer an easy way to connect with customers who are willing to provide their information. You can strategically place these forms on your site to collect email addresses, phone numbers, or mailing addresses to send out marketing material.
This could include special offers, coupons, company news, or simply a catalog. Don’t forget you can incentivize contact forms by turning them into a contest!
Testimonials
Forms can also be a good avenue for collecting reviews and testimonials. Embedding such a form after the checkout process or sending a link to your testimonial form via email can be a great way to encourage customers to leave a review.
These testimonials are excellent for gathering feedback and improving your operation. They can also be used in marketing material and on social platforms.
Order Forms
Some essential forms like account registration, checkout screens, and order forms may not have crossed your mind. While these are likely handled by other applications on your website, they can also be created with forms.
Simple order forms can allow customers to fill out what they wish to order along with their shipping and contact information to receive a quote. These can be programmed with dropdown menus or simple message fields with instructions.
Employee Applications
Employee applications are another great example of a common form. To make an application form, you can include fields for basic information like name and contact info, along with others for work experience, education, and qualifications.
You can also add a file upload field for a resume or CV. This can be much easier to manage than sifting through unstructured emails or paper copies.
Conclusion
Understanding and connecting with your customers is easier said than done. Especially in the world of eCommerce, where you lack face-to-face interactions. Therefore, you’ll likely need a way to collect feedback, marketing leads, and other essential information. Forms offer an excellent way to do this.
To recap, you can easily create a form in WordPress when you follow these steps:
- Install Ninja Forms.
- Design your form.
- Embed a form on your website.
- Review submission results.
WP Engine is known for fast and reliable hosting services. If you’re struggling to implement forms on your site, we also offer WordPress support with our managed hosting plans.